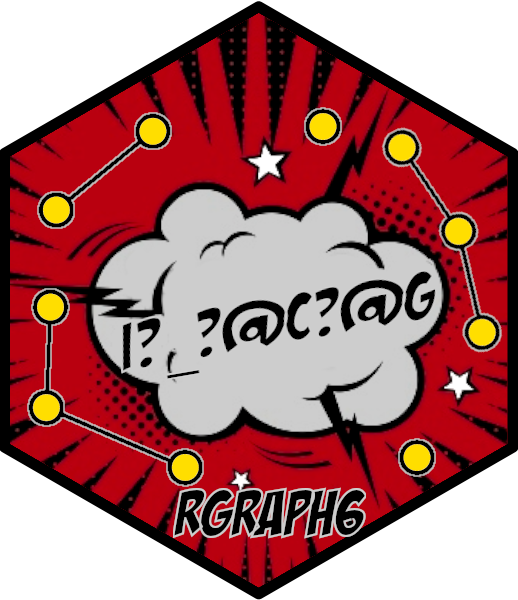
Create edgelist matrices from 'graph6', 'sparse6', or 'digraph6' symbols
Source:R/edgelist_from_text.R
edgelist_from_text.Rd
Create edgelist matrices from 'graph6', 'sparse6', or 'digraph6' symbols
Details
If object
contains 'graph6' or 'digraph6' symbols, which are in
fact encoded adjacency matrices, the function will return corresponding
edgelist matrices creating temporary igraph objects internally.
Examples
# Graph6 symbols
sampleg6
#> [1] "CR" "CJ" "CN" "F_?xo" "F@h^_" "F@Miw" "Hr\\~~~~"
#> [8] "H}u|z|~" "Ht\\~~z~"
edgelist_from_text(sampleg6)
#> [[1]]
#> [,1] [,2]
#> [1,] 1 3
#> [2,] 2 4
#> [3,] 3 4
#>
#> [[2]]
#> [,1] [,2]
#> [1,] 2 3
#> [2,] 2 4
#> [3,] 3 4
#>
#> [[3]]
#> [,1] [,2]
#> [1,] 1 4
#> [2,] 2 3
#> [3,] 2 4
#> [4,] 3 4
#>
#> [[4]]
#> [,1] [,2]
#> [1,] 1 2
#> [2,] 3 6
#> [3,] 3 7
#> [4,] 4 6
#> [5,] 4 7
#> [6,] 5 6
#> [7,] 5 7
#>
#> [[5]]
#> [,1] [,2]
#> [1,] 1 5
#> [2,] 1 7
#> [3,] 2 6
#> [4,] 2 7
#> [5,] 3 4
#> [6,] 3 5
#> [7,] 3 7
#> [8,] 4 6
#> [9,] 4 7
#> [10,] 5 6
#>
#> [[6]]
#> [,1] [,2]
#> [1,] 1 6
#> [2,] 2 7
#> [3,] 3 4
#> [4,] 3 5
#> [5,] 3 6
#> [6,] 4 5
#> [7,] 4 7
#> [8,] 5 6
#> [9,] 5 7
#> [10,] 6 7
#>
#> [[7]]
#> [,1] [,2]
#> [1,] 1 2
#> [2,] 1 3
#> [3,] 1 7
#> [4,] 1 8
#> [5,] 1 9
#> [6,] 2 4
#> [7,] 2 5
#> [8,] 2 6
#> [9,] 2 7
#> [10,] 2 8
#> [11,] 2 9
#> [12,] 3 4
#> [13,] 3 5
#> [14,] 3 6
#> [15,] 3 7
#> [16,] 3 8
#> [17,] 3 9
#> [18,] 4 5
#> [19,] 4 6
#> [20,] 4 7
#> [21,] 4 8
#> [22,] 4 9
#> [23,] 5 6
#> [24,] 5 7
#> [25,] 5 8
#> [26,] 5 9
#> [27,] 6 7
#> [28,] 6 8
#> [29,] 6 9
#> [30,] 7 8
#> [31,] 7 9
#> [32,] 8 9
#>
#> [[8]]
#> [,1] [,2]
#> [1,] 1 2
#> [2,] 1 3
#> [3,] 1 4
#> [4,] 1 5
#> [5,] 1 6
#> [6,] 1 7
#> [7,] 2 3
#> [8,] 2 4
#> [9,] 2 5
#> [10,] 2 8
#> [11,] 2 9
#> [12,] 3 6
#> [13,] 3 7
#> [14,] 3 8
#> [15,] 3 9
#> [16,] 4 5
#> [17,] 4 6
#> [18,] 4 7
#> [19,] 4 8
#> [20,] 4 9
#> [21,] 5 6
#> [22,] 5 7
#> [23,] 5 8
#> [24,] 5 9
#> [25,] 6 7
#> [26,] 6 8
#> [27,] 6 9
#> [28,] 7 8
#> [29,] 7 9
#> [30,] 8 9
#>
#> [[9]]
#> [,1] [,2]
#> [1,] 1 2
#> [2,] 1 3
#> [3,] 1 4
#> [4,] 1 7
#> [5,] 1 8
#> [6,] 1 9
#> [7,] 2 5
#> [8,] 2 6
#> [9,] 2 7
#> [10,] 2 8
#> [11,] 2 9
#> [12,] 3 4
#> [13,] 3 5
#> [14,] 3 6
#> [15,] 3 7
#> [16,] 3 8
#> [17,] 3 9
#> [18,] 4 5
#> [19,] 4 6
#> [20,] 4 7
#> [21,] 4 8
#> [22,] 4 9
#> [23,] 5 6
#> [24,] 5 7
#> [25,] 5 8
#> [26,] 5 9
#> [27,] 6 7
#> [28,] 6 8
#> [29,] 6 9
#> [30,] 7 9
#> [31,] 8 9
#>
# Sparse6 symbols
s6 <- c(":DgXI@G~", ":DgWCgCb")
edgelist_from_text(s6)
#> [[1]]
#> [,1] [,2]
#> [1,] 2 3
#> [2,] 2 4
#> [3,] 3 4
#> [4,] 1 5
#> [5,] 2 5
#> [6,] 3 5
#> [7,] 4 5
#> attr(,"gorder")
#> [1] 5
#>
#> [[2]]
#> [,1] [,2]
#> [1,] 2 3
#> [2,] 1 4
#> [3,] 2 4
#> [4,] 3 4
#> [5,] 1 5
#> [6,] 2 5
#> [7,] 3 5
#> [8,] 4 5
#> attr(,"gorder")
#> [1] 5
#>
# Digraph6 symbol
d6 <- "&N????C??D?_G??C?????_?C_??????C??Q@O?G?"
edgelist_from_text(d6)
#> [[1]]
#> [,1] [,2]
#> [1,] 2 13
#> [2,] 4 1
#> [3,] 4 3
#> [4,] 4 10
#> [5,] 5 3
#> [6,] 6 7
#> [7,] 8 10
#> [8,] 9 10
#> [9,] 9 13
#> [10,] 12 13
#> [11,] 13 14
#> [12,] 14 2
#> [13,] 14 9
#> [14,] 14 11
#> [15,] 15 9
#>